Creating KDE Applications: C++ Basics
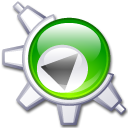
Introduction
This article explains the basics of C++; In this step, we continue with the basics about C++; control structures, functions and variables. You can safely skip this part if you already have some programming experience.
Variables
Variables act as a storing place for data. A value can be used in a formula, or to store the result of an mathematic operation. It's like Math, but fortunately C++ allows you to use longer names instead of X, Y and Z.
The following code structure should look a bit familiar already. A lot of the basics were explained in the chapter about compiling.
- A brief example of the use of variables:
#include <iostream> using namespace std; int main() { int firstValue = 4; int secondValue = 5; int sumValue; sumValue = (firstValue + secondValue); cout << "The sum is: " << sumValue << endl; }
The "int firstValue" part declares the integer-type variable called "firstValue". Some memory is reserved to store the data. The value 4 is stored in the variable. Once a variable is declared, the compiler knows what kind of data is stored in the variable, and you don't have to repeat the data-type name each time.
You can compile the code with:
- Compile the code:
g++ -o example1 -c example1.cpp
And run it with:
- Run the program:
./example1
Declaration without values
You can also declare a variable without assigning a value to the variable. This happens with the sumValue variable in the previous example. In such situation, the value of the variable is undefined. It can be any value, depending on the previous value stored at the location of the variable in the system memory.
Data types
An integer uses 4-bytes to store numbers up to 2 million, but it cannot store any decimals. To store values like 20.4, use the "float" or "double" data type, so it becomes: "double firstValue = 4.0". There a few other data types, but the most important ones to know are:
- char, uses 1 byte to store a ASCII character (or -128 to 127).
- bool, uses 4 bytes to store a boolean value (only true or false).
- int, uses 4 bytes to store integers (a mathematical term for a number without decimals)
- unsigned int or uint, same as above, but the "unsigned" prefix causes the data type to accept positive numbers only. A normal int has a range of approx -2 million to 2 million, but the unsigned int has a range of 0 to approx 4 million. This also works for other data types; an "unsigned char" allows values from 0 to 255.
- float, uses 4 bytes to store a floating-point number (7 digits)
- double, uses 8 byts to store a double-precision floating-point number (15 digits)
Unfortunately, the data size for each variable is not constant for every platform and compiler. Only the "char" type is guaranteed to have the same size at each platform, the other data types can differ. 64-bit systems will likely use 8 bytes for each data type instead of 4.
A note for "smart" people
You may have noticed that the "bool" type uses 4 bytes to store a simple "on" or "off" state. This might sound inefficient, but it's not. The truth is: a 32-bit CPU has a strong preference to data blocks of 32 bit (4 byte). Some CPU's will always load the data as 4 byte chunks. If you choose to use a 2-byte variable, the next variable will be retrieved in two steps and combined later. In other words, never try to be efficient by using the smallest variable type possible. It's useless. Just use "int" and "double" where you can.
Functions
A different concept in each programming language is the use of functions. A function (or procedure) is a separate set of tasks that can be initiated when you like.
- A brief example of a function:
#include <iostream> using namespace std; void perform_calculation(int myFirst, int mySecond) { int sumValue = (myFirst + mySecond); cout << "The sum is: " << sumValue << endl; } int main() { int firstValue = 4; int secondValue = 5; perform_calculation(firstValue, secondValue); }
This program has two functions now; the main() function, and the perform_calculation() function. The program still starts at the main() function, but at the end of the main() function, the perform_calculation() function is called. The definition of the perform_calculation() function specified two arguments, and each caller needs to supply those arguments.
The values of firstValue and secondValue will be copied to the "stack frame" of the second function; hence the myFirst and mySecond variables contain the same data main() provided in the "function call".
Returning data
A function cannot only accept data, but it can also return data. You can do this by replacing the "void" keyword with the data-type you like to return.
- A function returning a value:
#include <iostream> using namespace std; int perform_calculation(int myFirst, int mySecond) { int sumValue = (myFirst + mySecond); return sumValue; } int main() { int firstValue = 4; int secondValue = 5; int sumValue; sumValue = perform_calculation(firstValue, secondValue); cout << "The sum is: " << sumValue << endl; }
In the function body, the "return" command instructs your system to return the given value and exit the function prematurely. In this example, the returned value is copied to the sumValue variable, and displayed later.
Declaration vs Definition
A function can only be called if the declaration is known to the compiler. If you like to move the function to a different location, keep a copy of the function declaration above the main() function. This promises the compiler it will eventually find the correct function definition.
- A function declaration:
void perform_calculation(int myFirst, int mySecond);
Note the declaration doesn't include a function body, and the line ends with a semicolon.
Control Structures
Control structures are special commands that alter the flow of the code. It allows the programmer to define a different flow for the program (or storyline if you like comparing to tales).
C++ has a few control structures, and they might look familiar for someone who wrote JavaScript or PHP code before. This is a very quick introduction, if you never wrote an application before, a good book with excersies might be helpful too.
The if statement
The if-statement allows the programmer to execute certain steps only if a condition is true. For example:
- The if statement:
int myValue = 3; if( myValue > 5 ) { cout << "It's larger then 5" << endl; } else if( myValue > 3 ) { cout << "It's larger then 3" << endl; } else { cout << "The value is quite small" << endl; }
This program displays "It's larger then 5", "It's larger then 3" or "The value is quite small". The if-statement doesn't always have to include the "else if" and "else" parts. You can omit those, but you can also add a new extra "else if" conditions.
The while statement
The while statement causes a block of code to repeat itself until the given expression is "false".
- The while statement:
int value = 10; while( value > 0 ) { cout << value << "..." << endl; value--; } cout << "Launch!" << endl;
This program displays 10..., 9..., and finally 2..., 1..., Launch! The code in the while body repeats itself while the value is greater then 0. The "value--" line is a short notation to decrement the value by one (substract 1). BASIC programmers would likely write "value = value - 1".
The for statement
A simular approach can be taken with the for-statement. Think of the for-statement as a controlled version of while-statement.
- The for statement:
int value; for( value = 10; value > 0; value--) { cout << value << "..." << endl; } cout << "Launch!" << endl;
The for-statement has three parts;
- The first section initializes the value you want to use.
- The second section contains the testing-condition like the one used in the while-statement.
- The third section is called each time the loop repeats.
The switch-statement
The switch-statement is a sort-of "fall through" system. You define different cases, and if one of them is true, all code that follows will be executed. This includes code from the next case blocks. The system will end the switch-code prematurely if it encounters a break statement.
- The switch statement:
int value = 4; switch( value ) { case 1: cout << "one" << endl; break; case 2: cout << "two" << endl; break; case 3: cout << "three" << endl; break; case 4: cout << "four" << endl; break; default: cout << "I don't reconize that number" << endl; } switch( value ) { case 1: case 2: case 3: cout << "The value is 1, 2 or 3" << endl; break; case 4: cout << "The value is 4" << endl; }
This statement is sometimes used to replace a long list of if-else code. Unfortunately, it can only be used for numbers.
That's it for now. To learn more about the basic syntax of C++, please look through some online tutorials. There are plenty of them.
Related articles
External links
blog comments powered by Disqus